I am writing some tests for a project I am working on called Green Fee Broker.
The first thing I am doing is mocking the business layer for the WCF service that I am writing so I can inject it into the service’s constructor and write the service while isolating dependencies:
MockRepository mockRepository = new MockRepository();
IBookingManager manager = mockRepository.DynamicMock<IBookingManager>();
Next, I set an expectation that CreateTeeTime is called on the manager mock, which is injected into the service constructor:
Expect.Call(manager.CreateTeeTime(course, DateTime.Now)).Return(teeTimeStub);
Finally, I ReplayAll to record the mock, call the service and assert that I am getting back a TeeTime object:
mockRepository.ReplayAll();
BookingService service = new BookingService(manager);
TeeTime teeTime = service.CreateTeeTime(course, DateTime.Now);
Assert.IsTrue(teeTime.ConfirmationNumber == "ABC123");
Unfortunately, there is a bug in the line of code that sets up the expectation. With Rhino Mocks, for an expected behavior to be a match, the parameters must be an exact match. Notice the second parameter uses DateTime.Now. This means that the expectation will have a different parameter than the actual call, even if it is just a few milleseconds of a difference. As a result, the assertion will fail because the return is null.
The solution is to add the IgnoreArguments method call on the expectation:
Expect.Call(manager.CreateTeeTime(course, DateTime.Now)).Return(teeTimeStub).IgnoreArguments();
The IgnoreArguments() call will ensure that the parameters are discarded completely in determining a match.
Now I am green, and good to go!
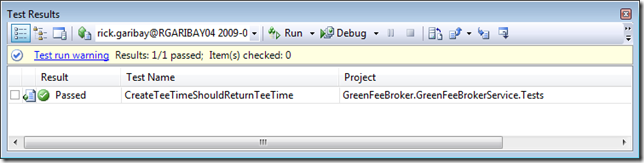